The Point of Pointers
02/22/21 08:07 Filed in: GO
Last time I learned about GO functions and parameters. Today, I want to learn about addresses and pointers in GO.
In this example from last time
func myFunction(word string, letter string, num int) {
}
and the call
myFunction("Test","A",3)
The three arguments "Test", "A", and 3 are passed by value to myFunction; that is, they are copied into the function. That means the original value isn't changed. Here is an example and its output.
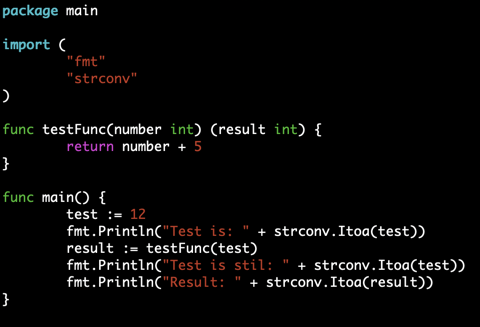
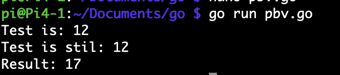
If you want to have the actual value changed, you can set test to result in main in this example. This is the "functional programming" way to do it. The other way, which GO supports, is through the use of address pointers.
GO is very much like "c" in some respects. In "c", as in GO, you use the & (ampersand) operator to get the memory address of something.
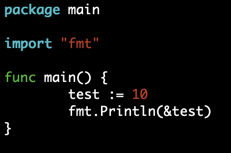
This is the address on my Raspberry Pi. Your address will be different.

Variables that store an address are known as pointers. (They point to a location in memory).
In order to declare a pointer variable you need to specify the type of the value stored in the specified memory. The declaration syntax becomes:
var pointer *type
For example:
var aPointer *int // a pointer to an int
var anotherPointer *string // a pointer to a string
The short declaration syntax is:
pointer := &variable
Which is used like this:
var hello string
pointerToHello := &hello
Here is an example of its use:
: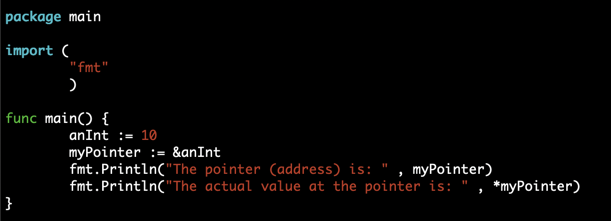

This is known as dereferencing a pointer.
Dereferencing can be used on the left side of a statement to change the value stored at the pointer (not the pointer itself). Assuming the above example,
&myPointer = 20
would change the value of anInt to 20. If you omit the &,
myPointer = 20
Would change the memory address stored in myPointer to 20. Unless you know what you are doing, in most programming languages, this will cause problems. In GO, you get an error message. Here is an expanded example and the error message.
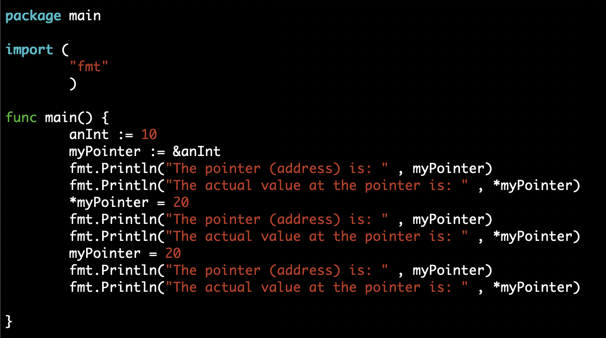

This is one reason GO is better (safer) than "c". In "c", it would let you assign the value and actually try to run the code which in most cases causes a crash.
So, in order to change the value of test within the testFunction in this example,
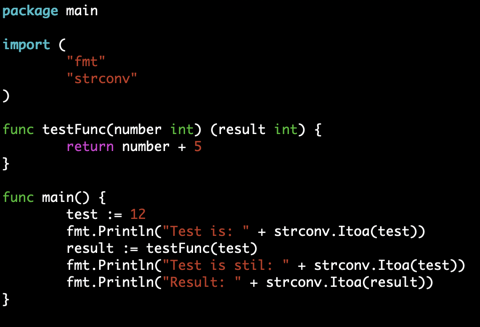
I can pass in a pointer as an argument. This is known as pass by reference.

When I do this, I don't need to return a separate value. This simplifies the code, but it really isn't "functional programming".
Pointers are very powerful, but can be difficult to learn and understand at first. They can also result in problems, including program crashes and alway be used with great care.
That's it for today.
In this example from last time
func myFunction(word string, letter string, num int) {
}
and the call
myFunction("Test","A",3)
The three arguments "Test", "A", and 3 are passed by value to myFunction; that is, they are copied into the function. That means the original value isn't changed. Here is an example and its output.
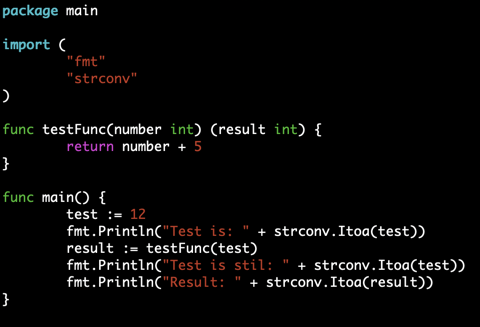
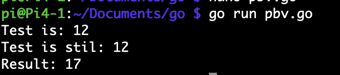
If you want to have the actual value changed, you can set test to result in main in this example. This is the "functional programming" way to do it. The other way, which GO supports, is through the use of address pointers.
Addresses and Pointers
GO is very much like "c" in some respects. In "c", as in GO, you use the & (ampersand) operator to get the memory address of something.
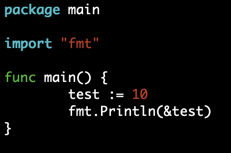
This is the address on my Raspberry Pi. Your address will be different.

Variables that store an address are known as pointers. (They point to a location in memory).
In order to declare a pointer variable you need to specify the type of the value stored in the specified memory. The declaration syntax becomes:
var pointer *type
For example:
var aPointer *int // a pointer to an int
var anotherPointer *string // a pointer to a string
The short declaration syntax is:
pointer := &variable
Which is used like this:
var hello string
pointerToHello := &hello
Here is an example of its use:
:
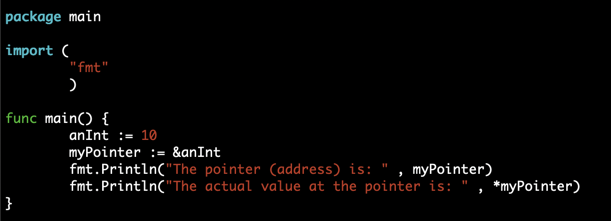

This is known as dereferencing a pointer.
Dereferencing can be used on the left side of a statement to change the value stored at the pointer (not the pointer itself). Assuming the above example,
&myPointer = 20
would change the value of anInt to 20. If you omit the &,
myPointer = 20
Would change the memory address stored in myPointer to 20. Unless you know what you are doing, in most programming languages, this will cause problems. In GO, you get an error message. Here is an expanded example and the error message.
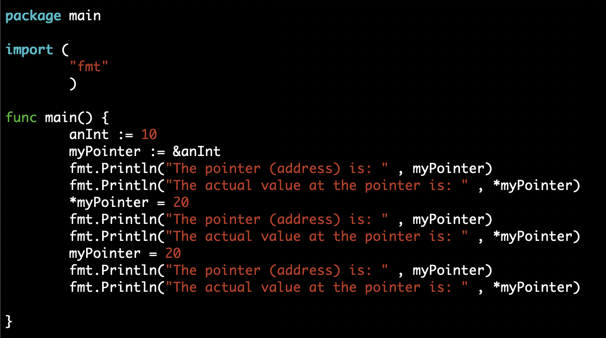

This is one reason GO is better (safer) than "c". In "c", it would let you assign the value and actually try to run the code which in most cases causes a crash.
Pointers and Functions
So, in order to change the value of test within the testFunction in this example,
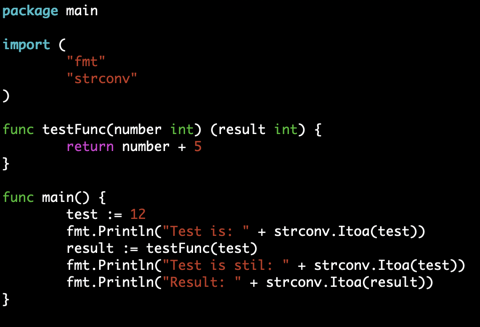
I can pass in a pointer as an argument. This is known as pass by reference.
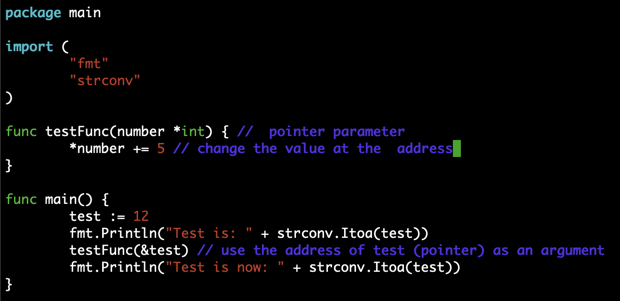

When I do this, I don't need to return a separate value. This simplifies the code, but it really isn't "functional programming".
Pointers are very powerful, but can be difficult to learn and understand at first. They can also result in problems, including program crashes and alway be used with great care.
That's it for today.