More On Printing & Functions
02/15/21 08:21 Filed in: GO
Today I'm going to cover formatting output in GO, as well as functions.
I've been writing small test programs as I learn and have been using the fmt.Println function to print values. This is fine but when printing irrational numbers and floating point values that have inherent rounding errors Println makes things messy. Here's an example.
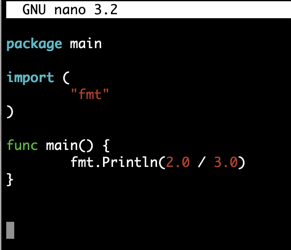

This is a typical programming issue. Luckily, GO has functions that are similar to c's printf and sprintf that allow you to format the output. Printf prints the value, Sprintf returns the formatted string. I'm not going to go into any detail since these work as expected if you are familiar with formatting in other languages. Here is the above example using both.
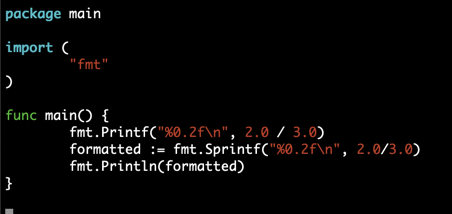
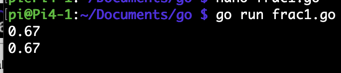
GO functions are blocks of reusable code, can accept arguments via parameters, and return values. The simplest function has the syntax:
func name() { // where name is the name of your function
}
Function parameters require type annotations, so the syntax becomes:
func name(p1 type, p2 type { //where p1, p2, etc are your parameter names and type is the type of parameter
}
For example:
func myFunction(word string, letter string, num int) {
}
Calling a function is as simple as using the function name and passing any arguments:
myFunction("Test","a",3)
A function can return a value. The function declaration must specify the return type using this syntax and use a return statement to pass a value of the specified type back to the caller.
func name() type {
return valueOfType
}
GO allows a function to return multiple values. Each return type needs to be listed in the declaration and enclosed in parentheses. Here is an example that returns three values.
func name() (int, string, bool) {
return 1, "TEST", false // example of the corresponding return statement.
}
I need to remember that the return values are not enclosed in parentheses.
You can also tag each return type in the declaration with a name:
func name() (name1 int, name2 string, name3 bool) {
return 1, "TEST", false // example of the corresponding return statement.
}
The tags are just that. They are not used in either the return or the call. As far as I can tell, the tags are just reminders or comments.
Any variables you declare within a function are local to the function. This works the way it does in most other languages.
In this example, I declare global variable a, a local variable a in the function and then print both from main.
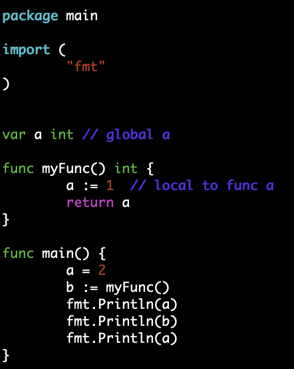

The local value shadows the global value, but does not change it. Generally speaking, you shouldn't shadow variable names since it can confuse thinking about what is happening in the code.
That's enough for today.
Printf and Sprintf
I've been writing small test programs as I learn and have been using the fmt.Println function to print values. This is fine but when printing irrational numbers and floating point values that have inherent rounding errors Println makes things messy. Here's an example.
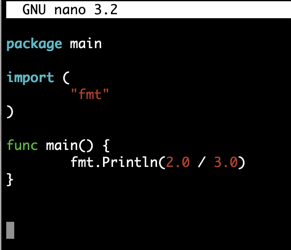

This is a typical programming issue. Luckily, GO has functions that are similar to c's printf and sprintf that allow you to format the output. Printf prints the value, Sprintf returns the formatted string. I'm not going to go into any detail since these work as expected if you are familiar with formatting in other languages. Here is the above example using both.
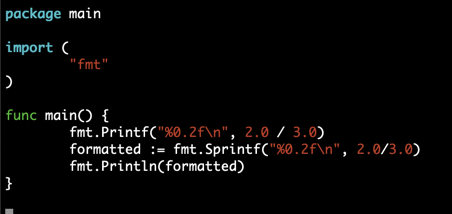
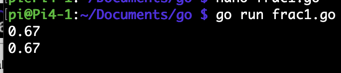
Functions
GO functions are blocks of reusable code, can accept arguments via parameters, and return values. The simplest function has the syntax:
func name() { // where name is the name of your function
}
Function parameters require type annotations, so the syntax becomes:
func name(p1 type, p2 type { //where p1, p2, etc are your parameter names and type is the type of parameter
}
For example:
func myFunction(word string, letter string, num int) {
}
Calling a function is as simple as using the function name and passing any arguments:
myFunction("Test","a",3)
Returning Values
A function can return a value. The function declaration must specify the return type using this syntax and use a return statement to pass a value of the specified type back to the caller.
func name() type {
return valueOfType
}
GO allows a function to return multiple values. Each return type needs to be listed in the declaration and enclosed in parentheses. Here is an example that returns three values.
func name() (int, string, bool) {
return 1, "TEST", false // example of the corresponding return statement.
}
I need to remember that the return values are not enclosed in parentheses.
You can also tag each return type in the declaration with a name:
func name() (name1 int, name2 string, name3 bool) {
return 1, "TEST", false // example of the corresponding return statement.
}
The tags are just that. They are not used in either the return or the call. As far as I can tell, the tags are just reminders or comments.
Scope In Functions
Any variables you declare within a function are local to the function. This works the way it does in most other languages.
In this example, I declare global variable a, a local variable a in the function and then print both from main.
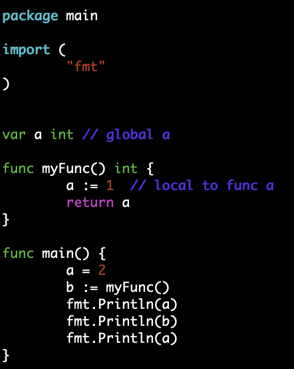

The local value shadows the global value, but does not change it. Generally speaking, you shouldn't shadow variable names since it can confuse thinking about what is happening in the code.
That's enough for today.