Packages
03/01/21 08:32 Filed in: GO
In one of the first posts I wondered why fmt.Println() used a lowercase fmt and an uppercase Println. In this post I dive into GO packages which answer the question.
Packages are bundled functions that can be imported into multiple programs. I've been using statements similar to this one to read packages into my programs
import (
"fmt"
"strings"
)
Where fmt and strings are packages. The GO compiler has a search path that it traverses to locate these packages. This path is known as the GO workspace. By default, the GO workspace resides in a directory in the user's home directory named and go. One thing to note is the go directory is not created by default, since it's considered a user directory. Within this directory are three subdirectories:
The src directory is the one of interest. It contains subdirectories named after specific packages which contain the source code files that make up the package.
Let's create a simple package. First, I need to set up the workspace.
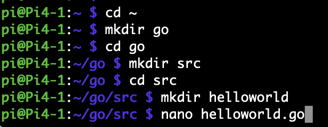
Here I change to my home directory, and then create the go and src directories. Within the src directory I create my package directory which I'm calling helloworld. Finally, I create a package file named helloworld.go which contains:
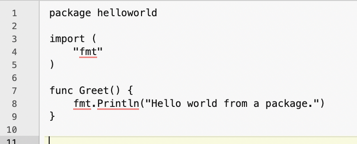
There are a couple of things to note. First, my package is named helloworld and can be anything, except main. Second, packages can import other packages. Here, I'm importing fmt. My package has a single function, Greet. The function name is capitalized because this function is exported. That's the GO convention. Finally, there is no main function, since this is a package or library.
I now have this directory structure:
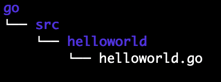
Now, I want to see if this set up works. I need a test program.
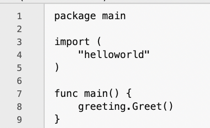
This is a normal program so its package is main and it has a main function. I don't import fmt, but instead import my package helloworld, which in turn imports fmt. On line 8 I call my Greet function that resides in the helloworld package.
Let's run it.

It works!
GO has some naming conventions I need to follow when dealing with packages.
If a package exports a constant, the constant name must be capitalized. In a normal program the syntax of a constant is:
const name type = value
For example:
const length int = 3
But if I want to export length from my package it would be:
const Length int = 3
And referenced from my main program as:
mypackage.Length
Also, I need to remember constants need to be declared outside of any functions in order to be exportable.
Packages can be nested. That is, within the package directory in src, we can have other packages. Here is my example.
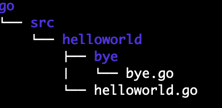
I've created a package, bye and a package file, bye.go within the helloworld package. In order to import this new package, the syntax of the import statement is:
import (
"helloworld/bye"
)
Essentially, you specify the path to the package directory. If the bye package contains a function Goodbye, I would call it with:
bye.Goodbye()
And not:
helloworld/bye.Goodbye()
There's more to GO packages, and I'll tackle those topics next time.
Workspace
Packages are bundled functions that can be imported into multiple programs. I've been using statements similar to this one to read packages into my programs
import (
"fmt"
"strings"
)
Where fmt and strings are packages. The GO compiler has a search path that it traverses to locate these packages. This path is known as the GO workspace. By default, the GO workspace resides in a directory in the user's home directory named and go. One thing to note is the go directory is not created by default, since it's considered a user directory. Within this directory are three subdirectories:
- bin - executables
- pkg - compiled package code
- src - source code
The src directory is the one of interest. It contains subdirectories named after specific packages which contain the source code files that make up the package.
Packages
Let's create a simple package. First, I need to set up the workspace.
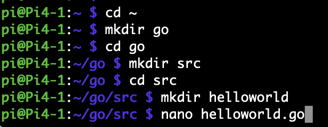
Here I change to my home directory, and then create the go and src directories. Within the src directory I create my package directory which I'm calling helloworld. Finally, I create a package file named helloworld.go which contains:
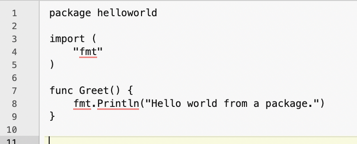
There are a couple of things to note. First, my package is named helloworld and can be anything, except main. Second, packages can import other packages. Here, I'm importing fmt. My package has a single function, Greet. The function name is capitalized because this function is exported. That's the GO convention. Finally, there is no main function, since this is a package or library.
I now have this directory structure:
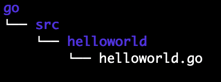
Now, I want to see if this set up works. I need a test program.
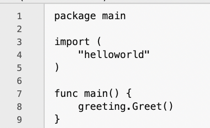
This is a normal program so its package is main and it has a main function. I don't import fmt, but instead import my package helloworld, which in turn imports fmt. On line 8 I call my Greet function that resides in the helloworld package.
Let's run it.

It works!
Naming Conventions
GO has some naming conventions I need to follow when dealing with packages.
- Package names are lowercase.
- Package names should be one word and abbreviated. If using two words, the package name is not separated and is all lowercase.
- Package names should avoid collisions with variable names.
- Functions that are exportable have capitalized names.
Exporting Constants
If a package exports a constant, the constant name must be capitalized. In a normal program the syntax of a constant is:
const name type = value
For example:
const length int = 3
But if I want to export length from my package it would be:
const Length int = 3
And referenced from my main program as:
mypackage.Length
Also, I need to remember constants need to be declared outside of any functions in order to be exportable.
Nesting Packages
Packages can be nested. That is, within the package directory in src, we can have other packages. Here is my example.
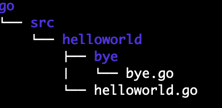
I've created a package, bye and a package file, bye.go within the helloworld package. In order to import this new package, the syntax of the import statement is:
import (
"helloworld/bye"
)
Essentially, you specify the path to the package directory. If the bye package contains a function Goodbye, I would call it with:
bye.Goodbye()
And not:
helloworld/bye.Goodbye()
There's more to GO packages, and I'll tackle those topics next time.