Variables and Variable Names
01/11/21 08:36 Filed in: GO
Variables
In GO variables are statically typed and use the syntax:
var name type [= initializer]
For example:
var total int
Or
var total int = 0.0
You can also assign multiple variables on the same line:
var volume, units = 10.2, "ml"
Which works as expected:
volume = 10.2
and units
is assigned a string value of "ml".You can also omit the type if you initialize the variable when you define it. The compiler will infer the type from the value assigned.
var length = 10.0 // a float64
var width = 3 // an int
var units = "inches" // a string
var isIt = false // boolean
Uninitialized variables get either a 0, 0.0, "", or false as default initializers.
Short Variable Declaration
GO has a simplified syntax for declaring and initializing a variable:
name := initializer
For example:
score := 12
And
var score = 12
Are identical.
Let's try a simple program.
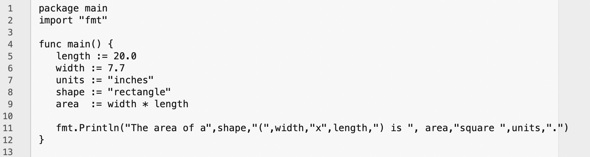
And, of course, it runs.

One interesting thing is that if you do this instead:
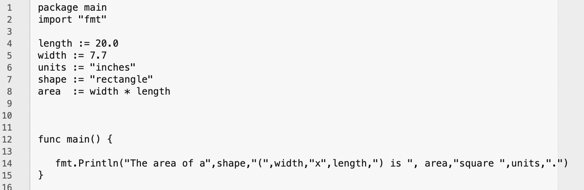
You get this when you run.

vars.go
is the name of my file. Line 4, column 1 has a syntax error. So, it seems that length := 20.0
is not a declaration? So, I tried this instead: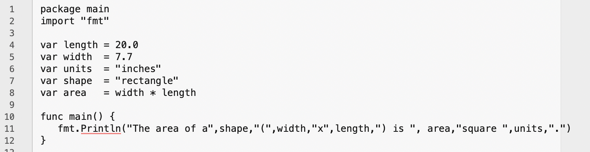
And this runs! So, it seems you can only use short declarations inside of function bodies. The
var
syntax is a bit more explicit, I guess, but I'm not thrilled by the inconsistency. Variable Names
Variable names start with a lowercase letter and can have any number of letters or numbers, unless the variable is exported and can be used outside the current package. Then, the variable starts with an uppercase letter. In GO camelcase is preferred as are abbreviations for common things (whatever "common" means). Here are some examples:
- stacks - local to the package
- Stacks - exported and accessible outside the current package.
- stacksOfCorn - local to the package
- StacksOfCash - exported
- min - instead of minimum
So, in line 11 above,
Println
is an exported function that is accessible outside the fmt
package.That's enough for today.