Things To Remember & For Loops
02/08/21 08:34 Filed in: GO
Things To Remember
As I mentioned before, in GO you can declare a variable two ways. First, explicitly by specifying a type:
var message string
Which declares a string called message. I need to remember that in GO the data type comes after the variable name. Although this is similar to many other languages, in GO, to me, this seems reversed. For some reason, I want to type:
var string message
Weird. I don't have this problem in other programming languages.
You can also declare a variable using the short declaration:
message := "This is a message."
Which dynamically types the variable as a string while initializing it. I need to remember that these two lines:
var string message
message := "This is a message."
would actually try to create two instances of the variable message which would cause problems or real errors depending on how and where this was done in the program. I think I need to be consistent and use either the first or second form throughout a program in order not to fall into this trap.
Another thing I need to remember is that when assigning multiple variables using the syntax:
x, y := 1, 2
y, z := 3, 4
The second line acts differently from the first. In the first line we are declaring two variables: x and y. We then assign 1 to x and 2 to y. In the second line, we do not declare a new variable y. In the second line, we assign 3 to the existing variable y, declare the variable z and assign 4 to it. Weird, but it makes sense.
For Loops
Just like many other programming languages, GO has a for loop. The GO syntax is pretty much the 'c' language syntax:
for x := 1; x < 10; x++ {
// stuff
}
You can also have a for statement in the form:
x := 0
for x < 10 {
// stuff
x++
}
Where the initialization of the control variable is externalized and the increment is handled in the loop. This can be useful, I think.
Just as in many other languages, GO also includes a break and continue statement. The break statement quits the current loop; continue skips the current iteration of the loop.
Let's write a quick test program.
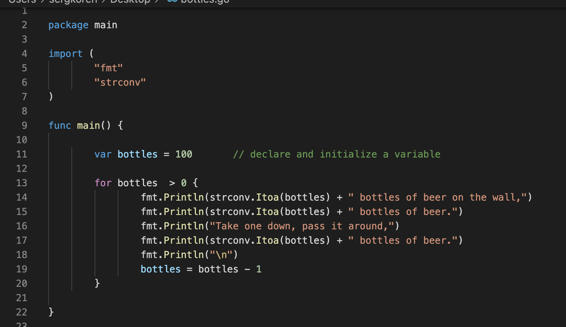
Here is the same thing in the 'c' style loop.
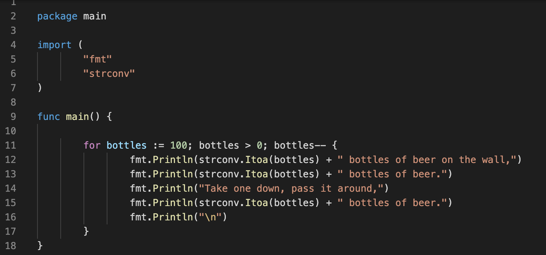
It's not perfect (it's brute force, needs a ton of refactoring, and its missing the last verse), but it tells me the loop works the way I expect it to.