Installation & Basic Commands
01/18/21 08:28 Filed in: GO
Last time, I looked at variables and variable naming. This time I'm going to install GO and then look at compiling and running programs.
Before I do that, however, I want to touch on two things I didn't cover last time.
I talked about types in GO. One thing I wanted to know was how to determine the type of a variable programatically. This is always a useful function to me (no pun intended). In GO, you can see the type of variable by using the TypeOf function. As you can see from the capitalized name this is an exported function. TypeOf is in the "reflect" package, so you have to import it. Let's print out the types of some variables to see the output in the GO Playground online.
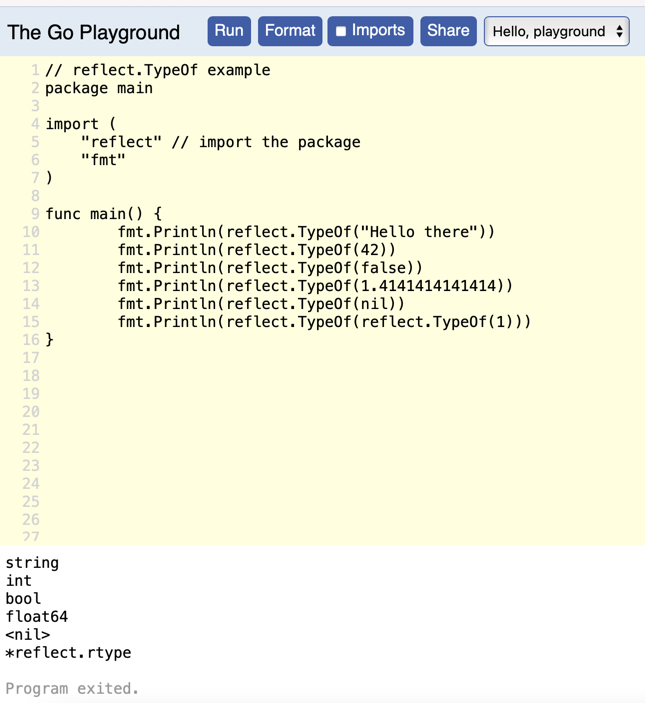
That's pretty much expected except the last "*reflect.rtype". That's printed by line 15 where I get GO to print the type of reflect.TypeOf() itself. The inner reflect returns int (but not an int) which is then passed to the outer reflect.TypeOf. So, it seems the reflect package has an rtype declared (reflect-type). I'm gong to assume the asterisk makes this a pointer to a reflect.rtype. (I don't know if GO has pointers, but given the syntax I'm guessing it does.).
Converting a variable of one type to a different type in GO is straight-forward if you are comfortable with other languages. You pass the variable you want to convert to a conversion function.
But, one nice thing I discovered writing an example was that the GO compiler flags imported packages that you don't use as errors. Most languages just let you import as many packages as you want. This little feature helps keep your source code clean.
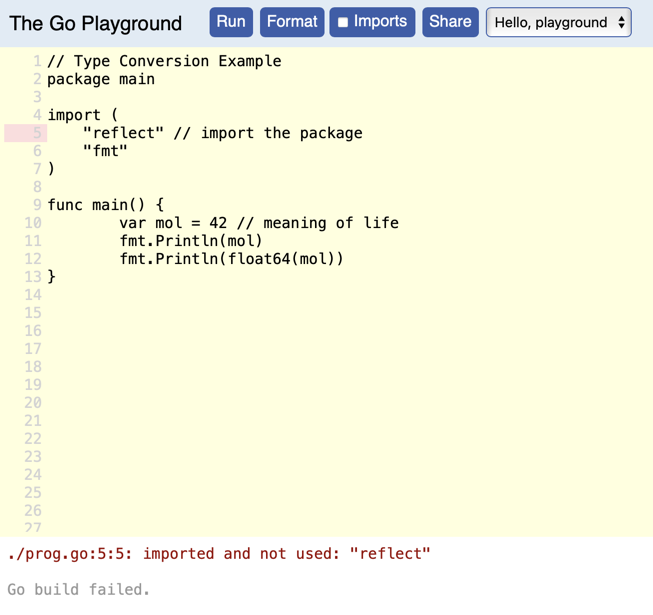
Fixing that…
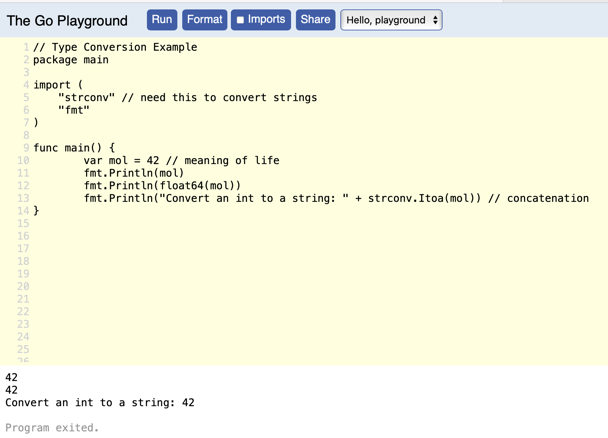
This example prints out an int, an int converted to a float64 and a string. In order to convert a number to a string you need to import the strconv package. The Itoa function converts an int to Ascii. This is pretty much just like you do it in "c". I haven't looked but I bet there is an Atoi function.
Using the web-based Playground is nice, but you wouldn't want to do anything serious with it. Let's install GO.
As usual, your source of truth about GO is https://golang.org. The site has a set of pre-built downloads and instructions for building it from source. I'm lazy, so I'm not going to try to build it on my MacBook M1. I'll install it on a Raspberry Pi. (Actually, I want to leant how to use GO, not how to build it from source. I'll do that later on the MacBook, but not cover it here.)
So, on my RaspberryPi Zero, I just issued the following command:
It took a couple of minutes and at the prompt to make sure it was installed, I printed the version.
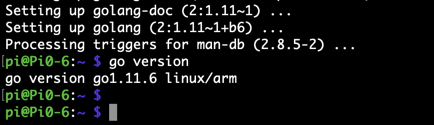
Let's compile something. First, I need a program to compile. This is my hello.go file.
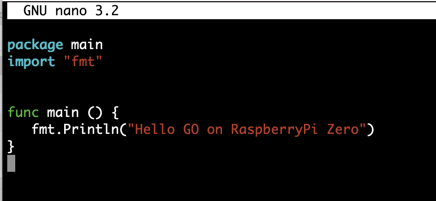
There are a couple ways to do this. First, you can build an executable. This is really just compiling to a binary. The syntax is:
You can then run the executable normally.
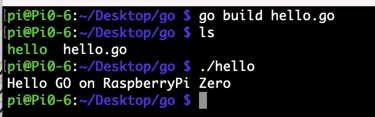
As you can see on the third line you the executable hello is green, and the source file hello.go is white.
You can also compile and run in one command using the syntax:
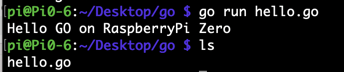
In this example the run command doesn't create an executable that is saved.
One final command that might be useful is gofmt. This command takes your source file and reformats it into a "standard" format. This command takes some optional arguments (switches) which you can find documented on https://golang.org/cmd/gofmt/. It prints its output to the default output (screen), but you can always redirect the output to a file.
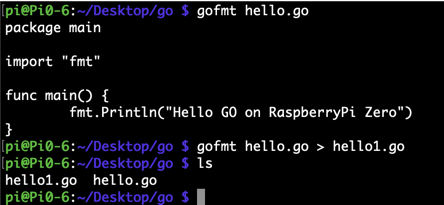
In my small example, it really didn't do anything.
In summary, I went through type conversion and finding the type of a variable using the reflect pacakge. I then covered the basics of installing GO and running programs on your device. Now that I have a working environment, I can learn the language, so I don't have to be stuck using Println for everything. In the next installment, I'll dive into some useful functions.
Stay tuned.
Before I do that, however, I want to touch on two things I didn't cover last time.
Find the Type of A Variable
I talked about types in GO. One thing I wanted to know was how to determine the type of a variable programatically. This is always a useful function to me (no pun intended). In GO, you can see the type of variable by using the TypeOf function. As you can see from the capitalized name this is an exported function. TypeOf is in the "reflect" package, so you have to import it. Let's print out the types of some variables to see the output in the GO Playground online.
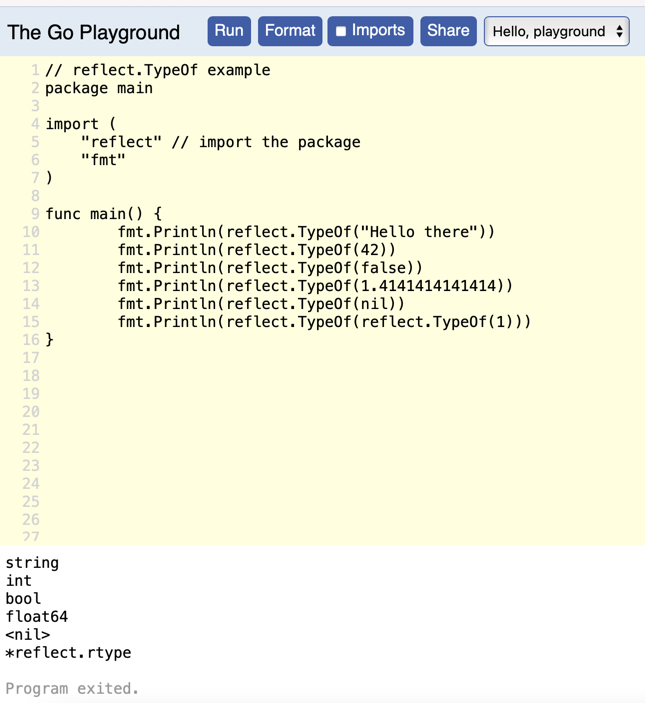
That's pretty much expected except the last "*reflect.rtype". That's printed by line 15 where I get GO to print the type of reflect.TypeOf() itself. The inner reflect returns int (but not an int) which is then passed to the outer reflect.TypeOf. So, it seems the reflect package has an rtype declared (reflect-type). I'm gong to assume the asterisk makes this a pointer to a reflect.rtype. (I don't know if GO has pointers, but given the syntax I'm guessing it does.).
Type Conversion
Converting a variable of one type to a different type in GO is straight-forward if you are comfortable with other languages. You pass the variable you want to convert to a conversion function.
But, one nice thing I discovered writing an example was that the GO compiler flags imported packages that you don't use as errors. Most languages just let you import as many packages as you want. This little feature helps keep your source code clean.
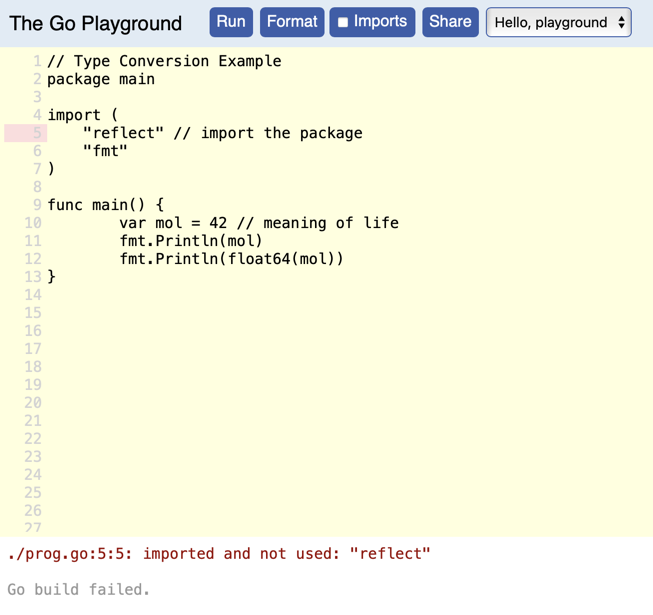
Fixing that…
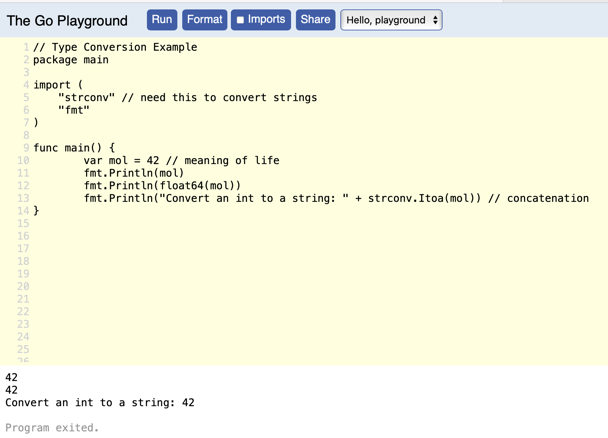
This example prints out an int, an int converted to a float64 and a string. In order to convert a number to a string you need to import the strconv package. The Itoa function converts an int to Ascii. This is pretty much just like you do it in "c". I haven't looked but I bet there is an Atoi function.
Using the web-based Playground is nice, but you wouldn't want to do anything serious with it. Let's install GO.
Installation
As usual, your source of truth about GO is https://golang.org. The site has a set of pre-built downloads and instructions for building it from source. I'm lazy, so I'm not going to try to build it on my MacBook M1. I'll install it on a Raspberry Pi. (Actually, I want to leant how to use GO, not how to build it from source. I'll do that later on the MacBook, but not cover it here.)
So, on my RaspberryPi Zero, I just issued the following command:
sudo apt-get install golang
It took a couple of minutes and at the prompt to make sure it was installed, I printed the version.
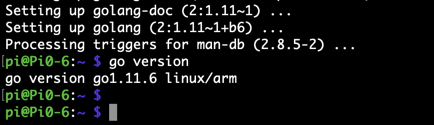
Compilation and Running
Let's compile something. First, I need a program to compile. This is my hello.go file.
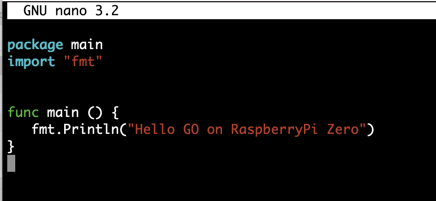
There are a couple ways to do this. First, you can build an executable. This is really just compiling to a binary. The syntax is:
go build filename
You can then run the executable normally.
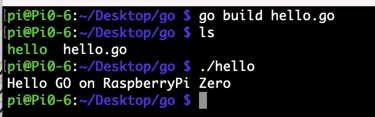
As you can see on the third line you the executable hello is green, and the source file hello.go is white.
You can also compile and run in one command using the syntax:
go run filename
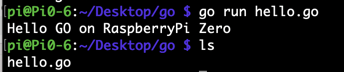
In this example the run command doesn't create an executable that is saved.
gofmt
One final command that might be useful is gofmt. This command takes your source file and reformats it into a "standard" format. This command takes some optional arguments (switches) which you can find documented on https://golang.org/cmd/gofmt/. It prints its output to the default output (screen), but you can always redirect the output to a file.
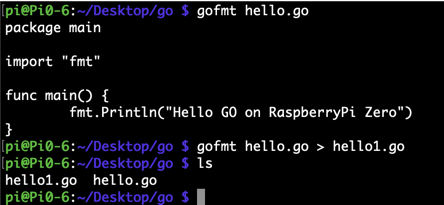
In my small example, it really didn't do anything.
In summary, I went through type conversion and finding the type of a variable using the reflect pacakge. I then covered the basics of installing GO and running programs on your device. Now that I have a working environment, I can learn the language, so I don't have to be stuck using Println for everything. In the next installment, I'll dive into some useful functions.
Stay tuned.