Arrays In GO
03/15/21 09:31 Filed in: GO
I'm done with GO packages for now. I want to get back to the core language. Today I learn about arrays.
Arrays in GO are declared using the syntax:
var arrayName [arraySize] type
So, for example:
var stateNames[50] string
Will create an array of 50 strings.
And
var tempsToday[100] int
Will create an array of 100 integers.
Assignment follows the same rules as other languages. GO, has the quick assignment that declares and assigns, however:
fruit := [3] string {"apple","banana","cherry"}
Which would declare and assign values to an array of 3 strings.
Indexing into an array is simply using the name and the index. fruit[2]. Assignment follows the same rule. Elements you don't attach values to are initialized to the 0 value for that type.
As in other languages, the type of array can be a more complex type and the value assigned has to match types. In all cases you'll get an error if you try to assign an incorrect value type. Here's a simple example.
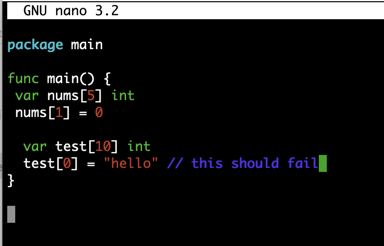
And the error I get when I run this.

What happens if I try to use an index out-of-range?
You get an interesting error if you try to use an index out of the range of the array:
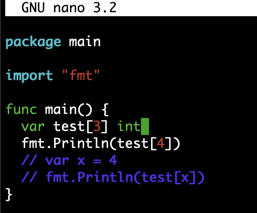
As expected, I get an error.

But I get a different error if I try to access the same array with a variable. That's the commented out section. Let's run that.
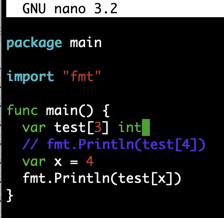
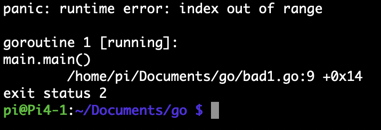
Weird. I get a runtime "panic". Most languages would give you the same error message. I think this is due to the differences in GO's build and run commands. I'm guessing the first error is built into the build command (compile time), the second in the run (runtime). So when I run go build bad1.go It first uses build and doesn't catch any compile time errors. GO then implicitly uses run (without a file save) to execute and catches the error. That's my guess.
So how do you loop over arrays? The same way you do in most other languages, with a for loop.
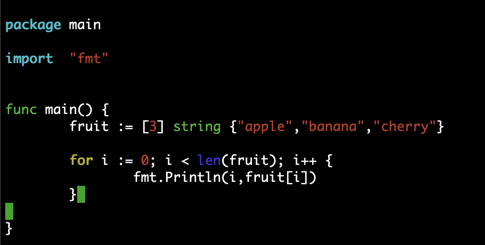
You can also use the range keyword in a for loop. This essentially generates an iterator for you. If you use this variant, you need to specify an index and value in the loop. The iterator will return both.
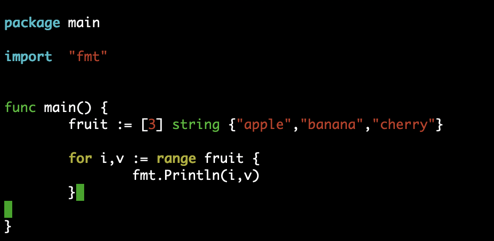
In both cases, this is the output:

If you're not interested in either the index or value, you can use the blank identifier:
for _, v := range fruit {
Again, this is similar to Swift's underscore.
Those are the basics of GO arrays.
Arrays
Arrays in GO are declared using the syntax:
var arrayName [arraySize] type
So, for example:
var stateNames[50] string
Will create an array of 50 strings.
And
var tempsToday[100] int
Will create an array of 100 integers.
Assignment follows the same rules as other languages. GO, has the quick assignment that declares and assigns, however:
fruit := [3] string {"apple","banana","cherry"}
Which would declare and assign values to an array of 3 strings.
Indexing into an array is simply using the name and the index. fruit[2]. Assignment follows the same rule. Elements you don't attach values to are initialized to the 0 value for that type.
As in other languages, the type of array can be a more complex type and the value assigned has to match types. In all cases you'll get an error if you try to assign an incorrect value type. Here's a simple example.
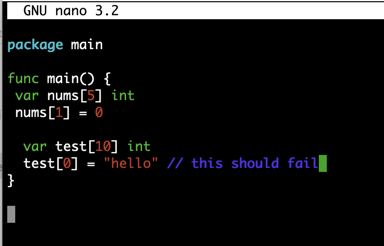
And the error I get when I run this.

What happens if I try to use an index out-of-range?
You get an interesting error if you try to use an index out of the range of the array:
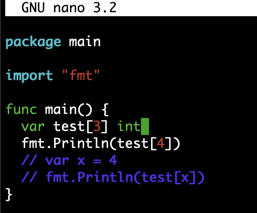
As expected, I get an error.

But I get a different error if I try to access the same array with a variable. That's the commented out section. Let's run that.
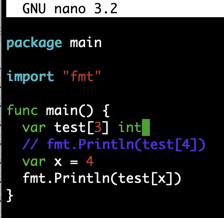
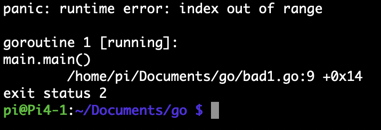
Weird. I get a runtime "panic". Most languages would give you the same error message. I think this is due to the differences in GO's build and run commands. I'm guessing the first error is built into the build command (compile time), the second in the run (runtime). So when I run go build bad1.go It first uses build and doesn't catch any compile time errors. GO then implicitly uses run (without a file save) to execute and catches the error. That's my guess.
So how do you loop over arrays? The same way you do in most other languages, with a for loop.
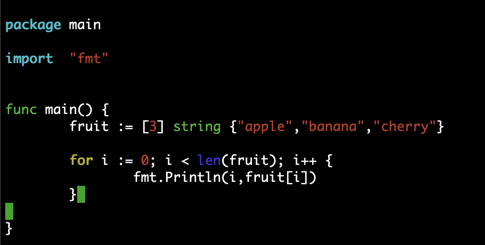
You can also use the range keyword in a for loop. This essentially generates an iterator for you. If you use this variant, you need to specify an index and value in the loop. The iterator will return both.
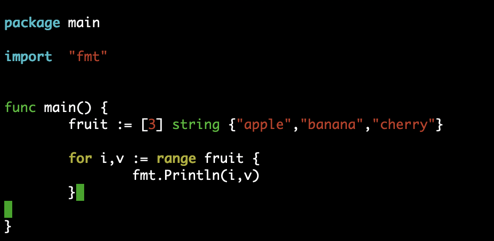
In both cases, this is the output:

If you're not interested in either the index or value, you can use the blank identifier:
for _, v := range fruit {
Again, this is similar to Swift's underscore.
Those are the basics of GO arrays.