Useful Functions
01/25/21 08:23 Filed in: GO
We've been using fmt.Println a lot. I want to use something else as well. Getting the current time (and date) is useful. In GO these are found in the time package which we'll have to import. Here is my test program.
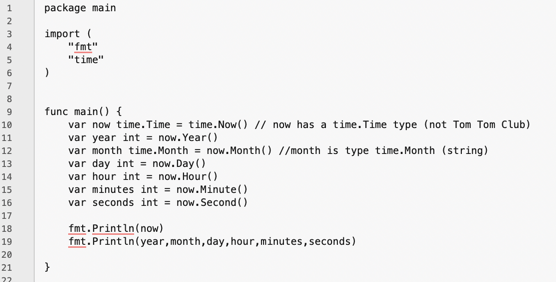
And this is the output:

The code is straight-forward apart from knowing the required types of time.Time and time.Month. So how do I get an integer month instead of a string? Well, the docs say that time.Month has an underlying type of int, so I should be able to coerce it.
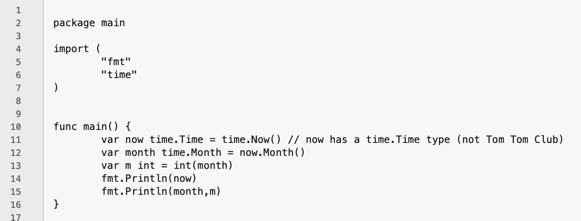

One other thing I tend to do a lot is replacing part of a string with another (I do a lot of text manipulation in my apps). In GO, there is a strings package which has a Replacer type. This can be used like this:
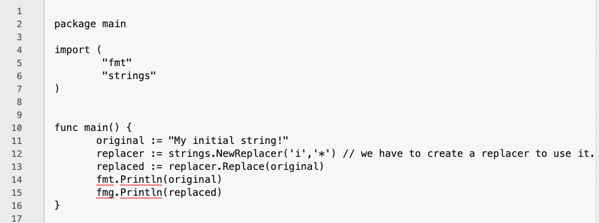
This causes an error saying I can't use i and * as runes (characters). So, I had to replace 'I' and '*' with "i", "*".
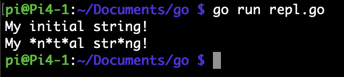
Line 12 looks like it's creating an instance of a replacer. I haven't run across it, but I'm going to assume GO uses class/objects or structs as well as functions (unless NewReplacer is an instatiator of a function). I'll figure it out later.
Another useful function to use is getting keyboard input from the user. In order to do this we need to import the bufio package which deals with buffered I/O. GO uses stdIn for input by default, which is the keyboard. This is familiar (to me), but we need to import the os package to deal with it. We can pass the stdIn stream to a buffered reader and assign the value to a variable. Let's try to read a string from the keyboard and echo it.
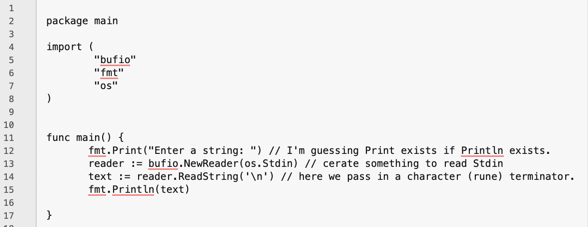
Huh? I get an error.

This is a very obtuse error message. It's pointing at line 14 which is the assignment to text. "multiple value" is a hint to me that the reader doesn't return a simple string, but multiple values? We talked about multiple return values previously. Let's try assigning to two values.
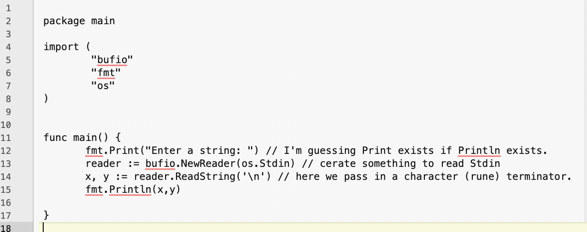
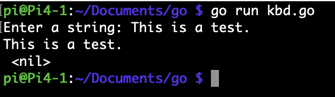
This runs! But what is that nil that is being returned? Checking the docs says that ReadString returns two values, the string and an error. Let's ignore the error for now. If we don't want to (or need to) use a return value we can use something called a blank identifier. This is the underscore (_). This is pretty much identical to Swift's _. It tosses the value into a bit-bucket and is ignored. So in lines 14, 15 we could have typed:
string, _ = reader.ReadString('\n')
fmt.Println(string)
That's enough for now.
Date & Time
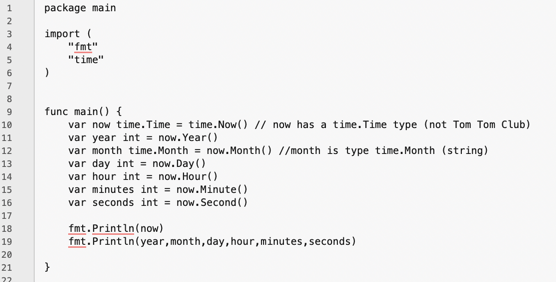
And this is the output:

The code is straight-forward apart from knowing the required types of time.Time and time.Month. So how do I get an integer month instead of a string? Well, the docs say that time.Month has an underlying type of int, so I should be able to coerce it.
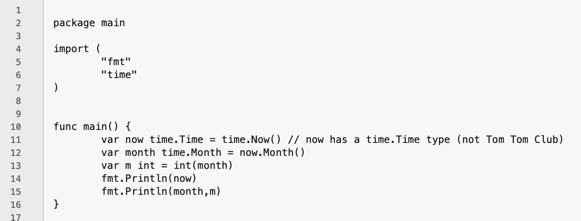

Substring Replacement
One other thing I tend to do a lot is replacing part of a string with another (I do a lot of text manipulation in my apps). In GO, there is a strings package which has a Replacer type. This can be used like this:
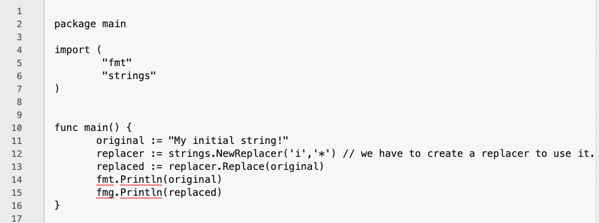
This causes an error saying I can't use i and * as runes (characters). So, I had to replace 'I' and '*' with "i", "*".
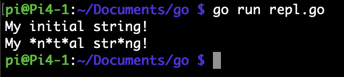
Line 12 looks like it's creating an instance of a replacer. I haven't run across it, but I'm going to assume GO uses class/objects or structs as well as functions (unless NewReplacer is an instatiator of a function). I'll figure it out later.
Reading User Input
Another useful function to use is getting keyboard input from the user. In order to do this we need to import the bufio package which deals with buffered I/O. GO uses stdIn for input by default, which is the keyboard. This is familiar (to me), but we need to import the os package to deal with it. We can pass the stdIn stream to a buffered reader and assign the value to a variable. Let's try to read a string from the keyboard and echo it.
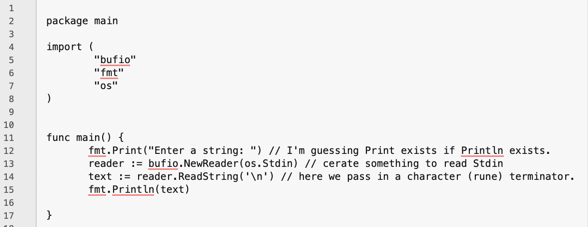
Huh? I get an error.

This is a very obtuse error message. It's pointing at line 14 which is the assignment to text. "multiple value" is a hint to me that the reader doesn't return a simple string, but multiple values? We talked about multiple return values previously. Let's try assigning to two values.
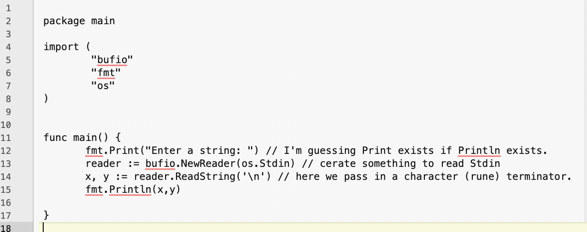
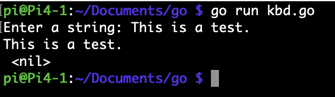
This runs! But what is that nil that is being returned? Checking the docs says that ReadString returns two values, the string and an error. Let's ignore the error for now. If we don't want to (or need to) use a return value we can use something called a blank identifier. This is the underscore (_). This is pretty much identical to Swift's _. It tosses the value into a bit-bucket and is ignored. So in lines 14, 15 we could have typed:
string, _ = reader.ReadString('\n')
fmt.Println(string)
That's enough for now.