Error Handling
06/29/21 09:04 Filed in: Lua
The last few posts have been about debugging in Lua. Today, I want to see how to handle errors in Lua code.
The last few posts have been about debugging in Lua. Today, I want to see how to handle errors in Lua code.
Lua doesn't have a way to handle or throw exceptions. That means, I have to handle errors the "simplistic" way of testing for an error code and handling it inside the test. Additionally, Lua also has something known as a protected call, or pcall. The syntax of pcall is:
ok result = pcall(function,[arguments])
Where function is a function to execute. [params] are a list of optional arguments to pass to function. ok is either true, or false depending on whether function encountered an error. result is either the error code, message, or actual result of function.
Here is a simple example:
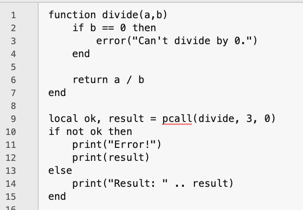
Here I use pcall to invoke the divide method which can either return an error when dividing by 0 or the result. I then test the returned values and handle them appropriately.
Is this better than just doing inline if statements, since we have inline if statements anyway? It's not as clean as try/catch blocks in other languages, but it does provide a consistent pattern.
As in other languages, you can use assert to terminate the program on a given condition. The syntax of assert is:
assert(condition, message)
Where condition is the condition which must be true to pass, and a message that is printed on the condition being false. Here's the divide function rewritten to use assert.
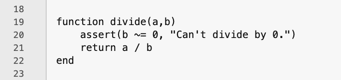
If a 0 is passed in as the second argument, the program will terminate with the message.
That's it for today.
pcall
Lua doesn't have a way to handle or throw exceptions. That means, I have to handle errors the "simplistic" way of testing for an error code and handling it inside the test. Additionally, Lua also has something known as a protected call, or pcall. The syntax of pcall is:
ok result = pcall(function,[arguments])
Where function is a function to execute. [params] are a list of optional arguments to pass to function. ok is either true, or false depending on whether function encountered an error. result is either the error code, message, or actual result of function.
Here is a simple example:
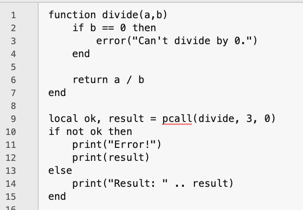
Here I use pcall to invoke the divide method which can either return an error when dividing by 0 or the result. I then test the returned values and handle them appropriately.
Is this better than just doing inline if statements, since we have inline if statements anyway? It's not as clean as try/catch blocks in other languages, but it does provide a consistent pattern.
assert
As in other languages, you can use assert to terminate the program on a given condition. The syntax of assert is:
assert(condition, message)
Where condition is the condition which must be true to pass, and a message that is printed on the condition being false. Here's the divide function rewritten to use assert.
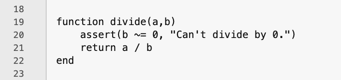
If a 0 is passed in as the second argument, the program will terminate with the message.
That's it for today.