Objects In Lua
02/23/21 08:08 Filed in: Lua
Today, I want to learn about OOP in Lua. Why OOP instead of functional programming? Because I still use OOP in many other languages and want to see how Lua handles it. Let's dive in.
Creating a Class
Lua is not an object-oriented (OO) language. Instead, I have to use Lua meta tables in order to create an OOP environment. First, I need a class. I can do this by using a normal table.
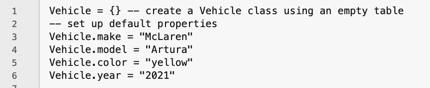
Creating and Using a Constructor
Now that I have a class defined, I need a constructor.

This is just a function that uses two parameters, self and object. The parameter self is used as a reference to the parent class/table Vehicle. The parameter object is the instance/table we want to create; that is, the initializer values. Line 10 needs a bit of explanation. The variable object is set to the passed in object or to a new empty table if object is not passed in. That is, the parameter object is optional and need not be passed into the constructor. Line 11 assigns self (my Vehicle class table) as the metatable to the new instance. Line 12 sets up the property chain. If I reference a property in my instance and it doesn't exist, a copy of the property from the class will be returned instead. Finally, line 13 returns the new instance.
Here is an example of using the constructor.

Line 17 creates a new instance of a Vehicle, this time a Ford. Line 18 adjusts the make (overriding the class's "McLaren". In this example, the constructor's line 18 sets the returned object to an empty table { }.
Here is an example of using the constructor and passing in initializer values.

Here, I'm creating an instance of an Acura, and initializing its make and color in the constructor, but leaving the year to the default in the Vehicle class (2021)
Now, let's try it out.
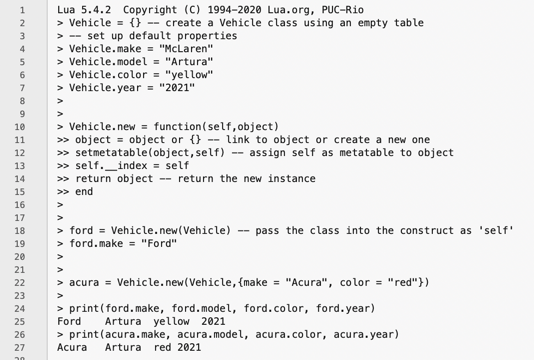
In lines 24 and 26 I print the instances and their properties. This proves this simple approach to classes and objects works. So far, I have classes, properties, and instances, but I have no methods.
Creating Methods
I can create a method on a Lua table, so I can create a method on my Vehicle class. Let's make my vehicle accelerate and decelerate. For that I need to keep track of its speed, so I'm going to add a property to the Vehicle class.
Vehicle.speed = 0 — initial speed is stopped
Now I can create two methods:
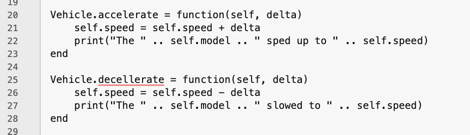
These are methods and belong to our class, Vehicle. That means I call it this way:

And this is what happens when it executes:

Creating Instance Methods
Creating an instance method is as simple as attaching a method to my instance object/table.
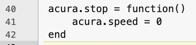
An Addition
Even though Lua doesn't natively support OOP, it's possible to use it. One thing that Lua does to help is it provides a special operator, the colon : which simplifies calls and makes them more OOP-like by returning the first parameter automatically. This is normally self.
So instead of calling:
Vehicle.accelerate(acura,10)
I can call
acura:accelerate(10)
and not have to make calls to the parent class.
That's it for today. I still need to learn about inheritance.