Welcome to Learning Lua
01/09/21 09:23 Filed in: Lua
Welcome to my Lua learning journey. I was originally going to include learning Lua as part of my getting up to speed on PICO-8, but I knew PICO-8 uses a subset of Lua and didn't want to conflate or confuse the two. I've decided to break Lua out separately.
So, what is Lua? Lua is an embeddable scripting language. It's used in a lot of games such as World of Warcraft and Roblox, in small devices and as a standalone language. And as I've mentioned PICO-8. It's small and can be compiled from source code by pretty much any C compiler and is actually just a C library.
Please note, this is not meant to be a set of tutorials, but more my notes and thoughts on learning Lua.
Lua comes preinstalled on RaspberryPi systems. I've decided to use it on my new Mac with the ARM M1 chip. A quick check in terminal shows that Lua is not installed (which isn't a surprise.) The main site for Lua is https://lua.org. I doubt there is a version that runs on the new system, so I decide to download and build from source. The webpage has a nice script on the right hand side under "Building".
Variables are straightforward. There are no real types in Lua. You just assign a value to a string.
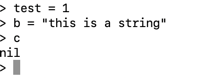
Referencing a variable (such as "c", above) returns nil. Unassigned variables return nil. Printing a variable is just as easy.
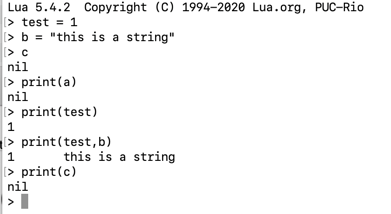
Lua has two types of comments: line and block. Line comments start with —

And block comments which are delimited by —[[ ]].
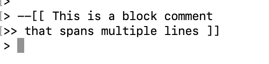
Lua uses implicit typing. Types are not declared, and you can reassign a different typed-value to a variable. The following is valid:
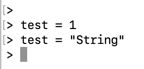
Lua values can be:
You can determine the type of a variable by using a built-in function called
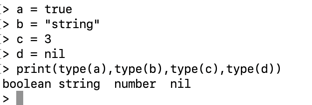
That's enough for now. Next, I'll dive into each of the value types.
Please note, this is not meant to be a set of tutorials, but more my notes and thoughts on learning Lua.
Installation
Lua comes preinstalled on RaspberryPi systems. I've decided to use it on my new Mac with the ARM M1 chip. A quick check in terminal shows that Lua is not installed (which isn't a surprise.) The main site for Lua is https://lua.org. I doubt there is a version that runs on the new system, so I decide to download and build from source. The webpage has a nice script on the right hand side under "Building".
curl -R -O http://www.lua.org/ftp/lua-5.4.2.tar.gz
tar zxf lua-5.4.2.tar.gz
cd lua-5.4.2
make all test
I copy and paste this into Terminal and everything runs fine. Now to install it rather than just testing the build, you have to run:
sudo make all install
The sudo is required since the command needs permission to write to the man directory. Everything runs fine and a quick check shows that Lua is now installed.
We're good to do. Let's dive right in.
Variables
Variables are straightforward. There are no real types in Lua. You just assign a value to a string.
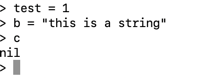
Referencing a variable (such as "c", above) returns nil. Unassigned variables return nil. Printing a variable is just as easy.
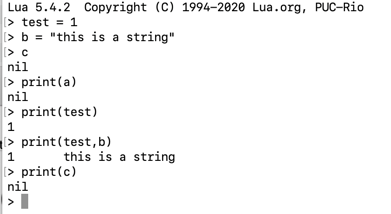
Lua has two types of comments: line and block. Line comments start with —

And block comments which are delimited by —[[ ]].
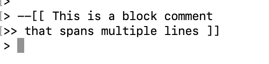
Data Types
Lua uses implicit typing. Types are not declared, and you can reassign a different typed-value to a variable. The following is valid:
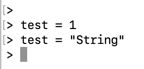
Lua values can be:
- nil - unknown or invalid
- String - array of characters in double-quotes
- Number - any real or decimal number
- Boolean - true or false
- Function - just what it sounds like
- Table - what other languages call dictionaries. Key-value pairs.
- Userdata - structures defined in "C".
- Thread - parallel code.
You can determine the type of a variable by using a built-in function called
type
. The function type
always returns a string.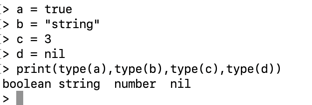
That's enough for now. Next, I'll dive into each of the value types.