Tables
02/02/21 08:16 Filed in: Lua
Today, I dive into something Lua calls a "table". This is a data structure, but I doubt it's a table in the SQL database definition.
Tables in Lua
Lua has a single built-in data structure it calls a table. Lua does not have built-in arrays, or dictionaries, just tables which can be used as these more conventional types. Lua's tables can even implement an OO environment in Lua, if desired. Interesting. Tables are a form of extended array that can take any type of index: numeric, string, or mixed–anything but nil. So, if all your indices are numeric, you end up with an array, if anything else, a dictionary. Lua table elements are initialized using the syntax:
variable = {}
which creates an empty table. Values are indexed using square brackets.
variable[index] = value
I'm using the term index to refer to both a numeric index, and a string key. You can mix index types in the same table, so the following example is valid.
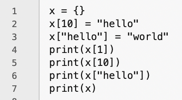
And this is the output when run.
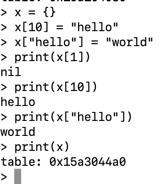
Unassigned values default to nil, as shown by printing x[1]. The interesting thing about tables, for me, is line 7 that I assumed would dump the entire table, but instead it prints table: followed by what looks like a memory address. This says, to me, that tables are stored by reference, not value. All other variables are stored by value in Lua. I guess I have to iterate over the table to print all of its elements.
Another interesting bit about tables is that if you use a string index/key, you can use a dot syntax to refer to an element:
x.hello is equivalent to x["hello"]. This doesn't work with a numeric index.
As in other languages, you can initialize values as part of the constructor:
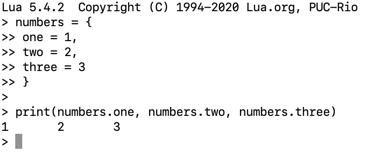
Tables as Arrays
Lua tables can implement arrays if the index is numeric-only. One thing that surprised me is that Lua indices start with 1 instead of 0, as in most other languages. In order to initialize an array with a constructor, you use a similar syntax to other programming languages. It's like the constructor above, but without the specified indices. Here is an example:
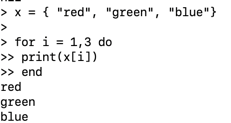
As you can see, arrays are 1-based instead of starting with 0. Because, as in the first example I showed that used x[10] = "hello", and didn't initialize indices 1 through 9, Lua arrays are sparse. The uninitialized values default to nil.
You can find the size (number of elements) of an array using the same # operator we use to find the length of a string. So in the previous example,
print(#x)
Would print 3. One strange thing about sparse arrays in Lua is that the # will quit counting if it encounters two consecutive nil values–but not always.
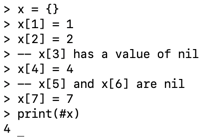
In this example, you get the wrong result. It prints 4 instead of 7. But in this example:
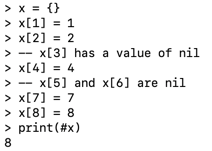
It prints the correct value of 8. Lua doesn't store this particular array as sparse. Weird. I don't think I want to dive into learning when an array is sparse, and when it is not. I'll just not use the # operator on arrays.
Multidimensional Arrays
Multidimensional arrays in Lua are created by creating an array and iterating over it to set each element to secondary array. You reference elements using consecutive indexes, [ ][ ]. Here is an example:
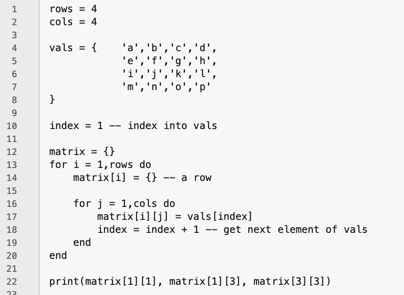
And the output:

This is a lot to take in, so I'm going to stop here for today.