Basic Animation
Now that we know how to load and save programs in PICO-8, let's do something that PICO-8 is "all about"–animation.
PICO-8 can animate at either 30, the default, or 60 frames per second (fps). We won't be doing anything that requires the faster frame rate so you can assume everything you see is being animated at 30fps.
Let's start with something simple. We know how to draw text on the screen, let's animate the text. Here is the program I used last time in my external editor.
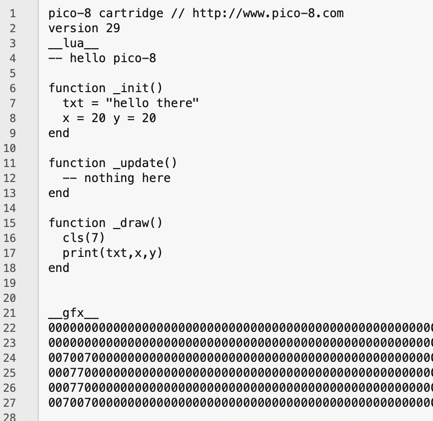
Our program is between lines 5 and 20. Don't touch the header or the __gfx__ area.
The __init() function is called once when the program starts. It's used to initialize the environment and game state. The _update function is called at 30 fps before the _draw function. This is where you update the game state, move characters, change sounds, and music. The _draw function is where you actually draw to the screen. The _update is split from the _draw because _drawing can take some time. PICO-8 will always do an _update, but may drop frames (_draw), if you do something really complex, in order to keep up. That is, logic is more important than drawing the screen.
Right now all our program does is print "hello there" at 30fps. It doesn't look like animation because the text doesn't move around, but it is animating. Let's move the text. We can do this by changing the text's location (x,y) in the _update function
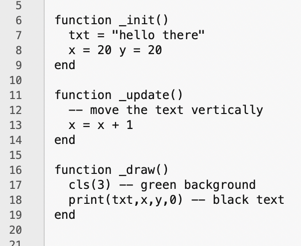
On line 13, I increment the value of X so the text will move left to right horizontally. Yes, I know the comment is wrong; it says vertically. I also changed the colors so the background is green (3) and the text is black (0), using the color table the PICO-8 site. When you run this, this is what you see:
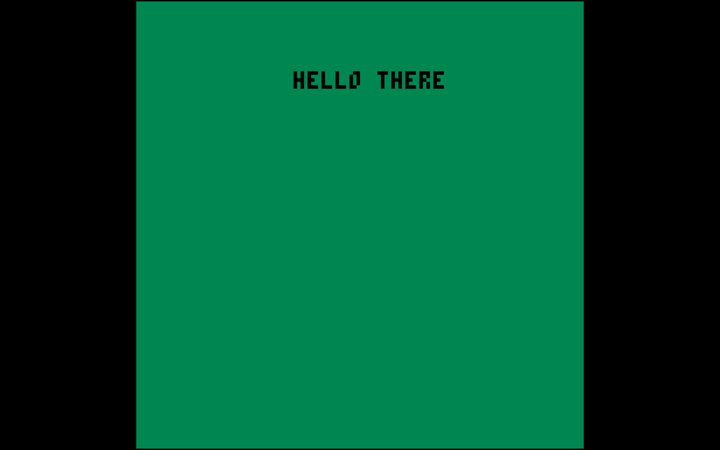
Except that the text animates. I don't feel like capturing videos and posting them. Run the program, you'll see it animates.
One thing you'll notice is the text animates off the right edge (and keeps going until you overflow x.) The PICO-8 resolution is 128x128 (big pixels) so we should do something when the text hits the edge. We'll keep it simple and only check the upper-left coordinate (x) of the text.
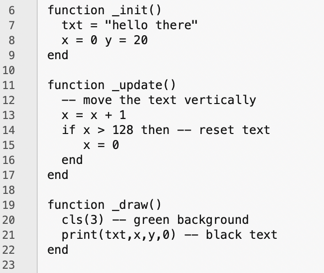
On lines 14-16, I add a Lua "if" statement. If the value of x hits 128, it resets to 0. I also changed line 8 to initialize x to 0 instead of 20. When you run this, the text slides off the right edge and then reappears on the left edge. Simple, but it works. I feel bad about the code. I want to make one more change.
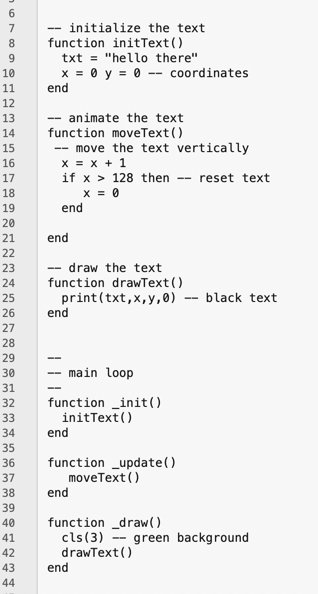
This looks like a lot more code. It's really not. I've moved all the bits into their own functions. Functions in PICO-8 pretty much work like other languages. Check out my Lua blog for more information on how functions differ. Why did I do this if it runs just like the previous version? This makes the code in the functions reusable and many small "atomic" functions are better than putting everything into the three game loop functions.
Animating text is fine, but let's draw our first graphics. I'll start simple: a pixel. In PICO-8.
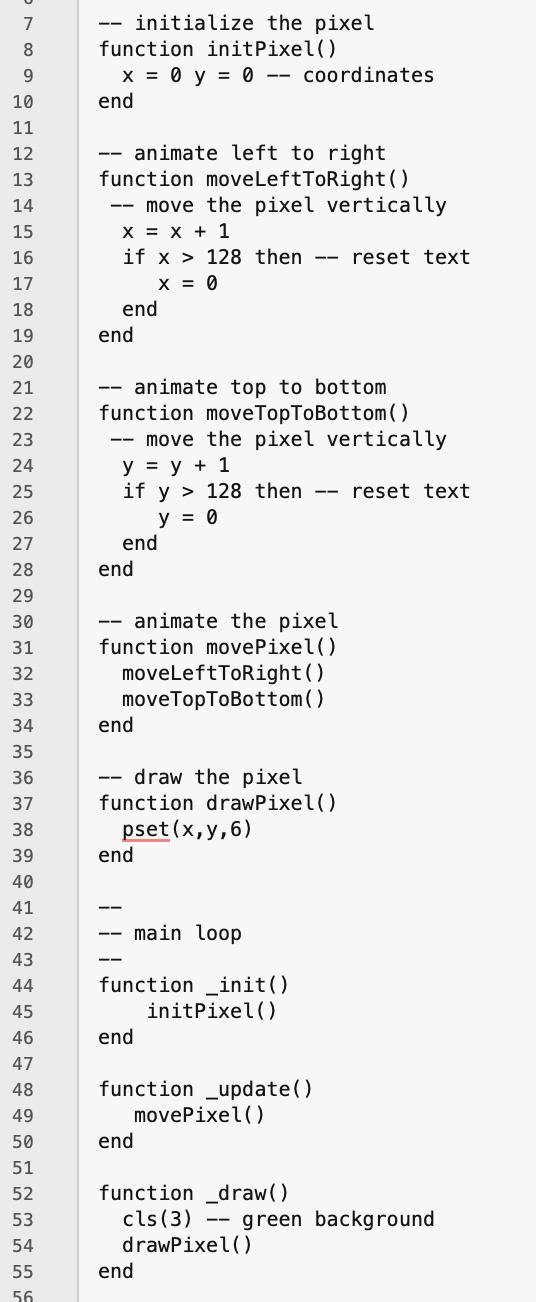
This is basically the same program, but I've gotten rid of the text and set up a pixel (which only has an x,y, and a color.) I've also refactored the code some more because I've added animating vertically. When you run this you get a pixel (dot) move from the upper left corner to the bottom right. The key line is 38.
pset(x,y,6)
PICO-8's pset command sets a pixel at the x,y coordinates using the color specified, in this case 6.
Now that we have this program animating other things are easy. To animate a circle, change line 38 to:
circ(x,y,r,c) — r îs the radius, c is the color
If you keep line 38 and add this between line 37 and 38 you get this when it runs:
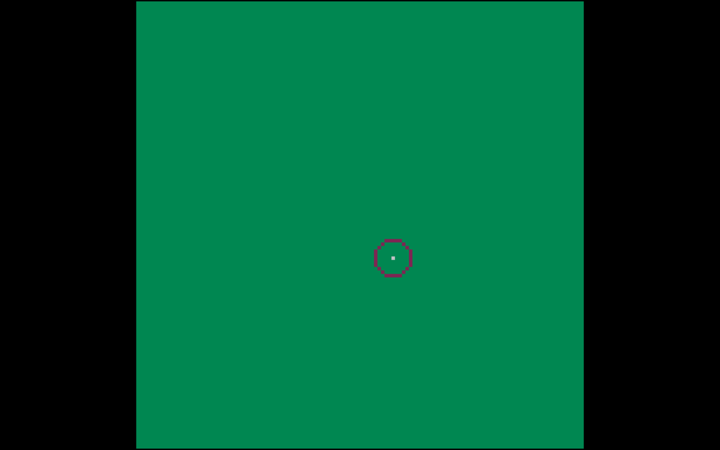
That's a little more interesting than text or a dot. To draw a filled circle, you use the circfill command.
circfill(x,y,r,c)
Rectangles are just as easy:
rect(x,y,x1,y1,c)
rectfill(x,y,x1,y1,c)
Where x,y is the upper left corner of the rectangle, and x1,y1 is the bottom right. Drawing a line follows the same pattern.
line(x,y,x1,y1,c)
Where x,y is the coordinate of one end of the line, and x1,y1 is the other end.
That's enough for now. I have the basics of animating text as well as basic shapes. Next time I'll dive into PICO-8's sprite editor and creating and animating sprites.
You can pull my cart here and run it if you want.
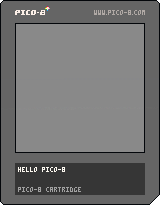
See you next time.
Let's start with something simple. We know how to draw text on the screen, let's animate the text. Here is the program I used last time in my external editor.
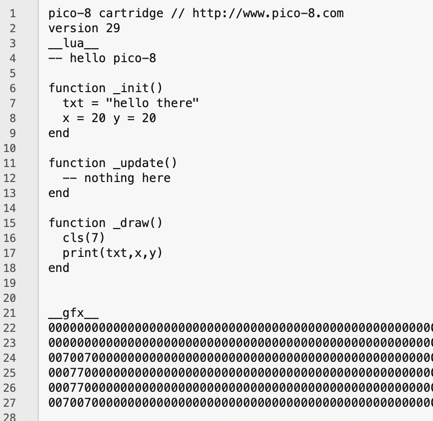
Our program is between lines 5 and 20. Don't touch the header or the __gfx__ area.
The __init() function is called once when the program starts. It's used to initialize the environment and game state. The _update function is called at 30 fps before the _draw function. This is where you update the game state, move characters, change sounds, and music. The _draw function is where you actually draw to the screen. The _update is split from the _draw because _drawing can take some time. PICO-8 will always do an _update, but may drop frames (_draw), if you do something really complex, in order to keep up. That is, logic is more important than drawing the screen.
Right now all our program does is print "hello there" at 30fps. It doesn't look like animation because the text doesn't move around, but it is animating. Let's move the text. We can do this by changing the text's location (x,y) in the _update function
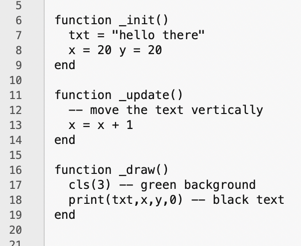
On line 13, I increment the value of X so the text will move left to right horizontally. Yes, I know the comment is wrong; it says vertically. I also changed the colors so the background is green (3) and the text is black (0), using the color table the PICO-8 site. When you run this, this is what you see:
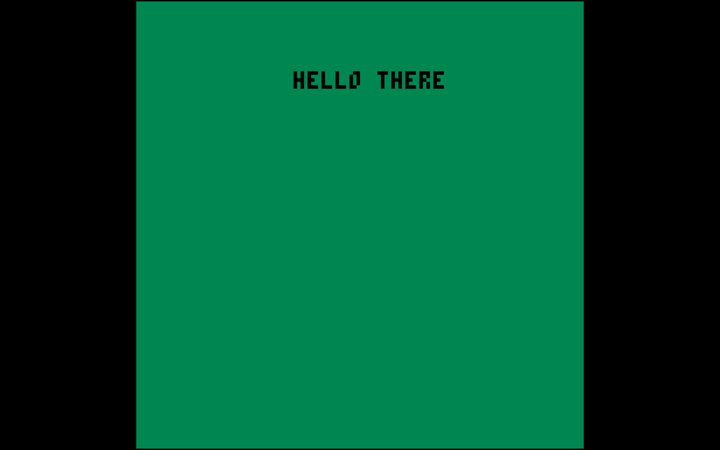
Except that the text animates. I don't feel like capturing videos and posting them. Run the program, you'll see it animates.
One thing you'll notice is the text animates off the right edge (and keeps going until you overflow x.) The PICO-8 resolution is 128x128 (big pixels) so we should do something when the text hits the edge. We'll keep it simple and only check the upper-left coordinate (x) of the text.
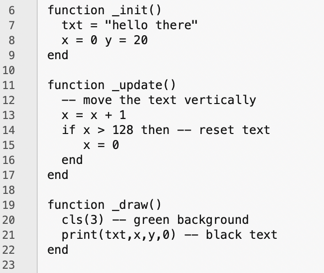
On lines 14-16, I add a Lua "if" statement. If the value of x hits 128, it resets to 0. I also changed line 8 to initialize x to 0 instead of 20. When you run this, the text slides off the right edge and then reappears on the left edge. Simple, but it works. I feel bad about the code. I want to make one more change.
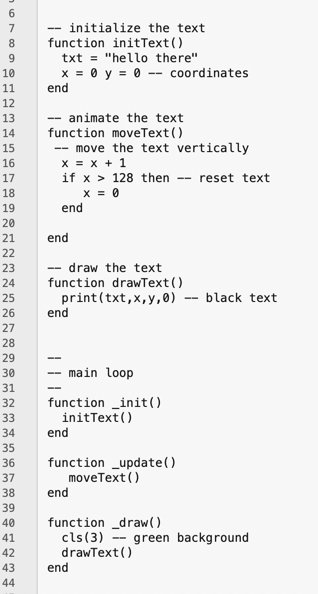
This looks like a lot more code. It's really not. I've moved all the bits into their own functions. Functions in PICO-8 pretty much work like other languages. Check out my Lua blog for more information on how functions differ. Why did I do this if it runs just like the previous version? This makes the code in the functions reusable and many small "atomic" functions are better than putting everything into the three game loop functions.
Basic Graphics
Animating text is fine, but let's draw our first graphics. I'll start simple: a pixel. In PICO-8.
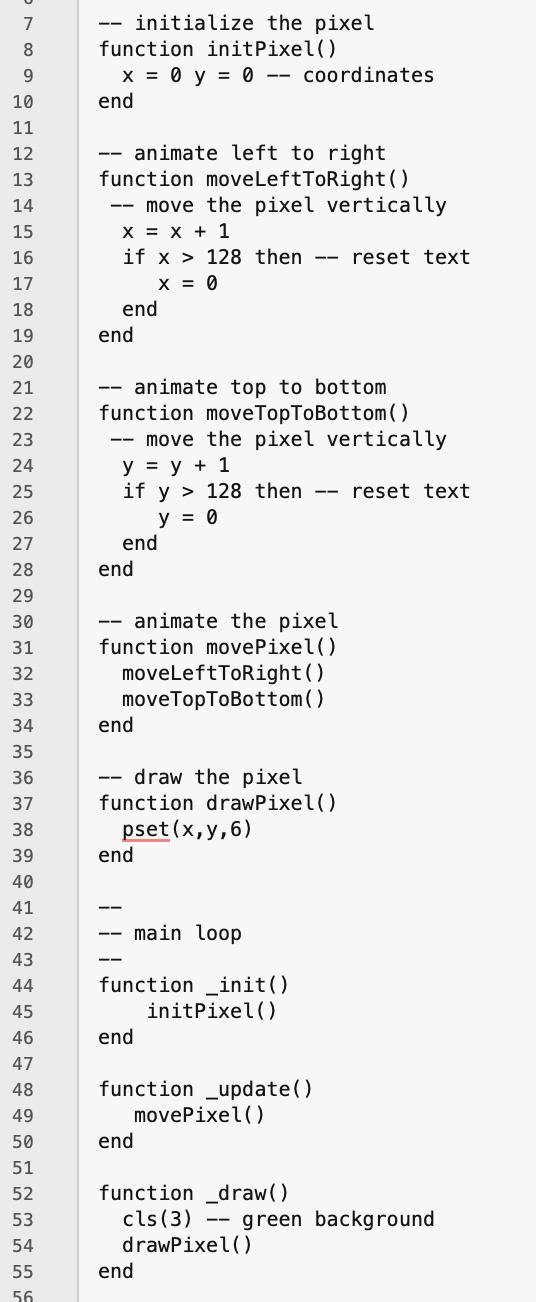
This is basically the same program, but I've gotten rid of the text and set up a pixel (which only has an x,y, and a color.) I've also refactored the code some more because I've added animating vertically. When you run this you get a pixel (dot) move from the upper left corner to the bottom right. The key line is 38.
pset(x,y,6)
PICO-8's pset command sets a pixel at the x,y coordinates using the color specified, in this case 6.
Now that we have this program animating other things are easy. To animate a circle, change line 38 to:
circ(x,y,r,c) — r îs the radius, c is the color
If you keep line 38 and add this between line 37 and 38 you get this when it runs:
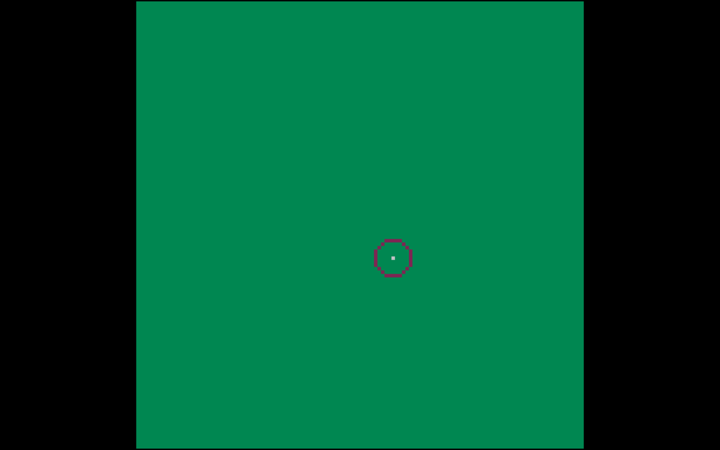
That's a little more interesting than text or a dot. To draw a filled circle, you use the circfill command.
circfill(x,y,r,c)
Rectangles are just as easy:
rect(x,y,x1,y1,c)
rectfill(x,y,x1,y1,c)
Where x,y is the upper left corner of the rectangle, and x1,y1 is the bottom right. Drawing a line follows the same pattern.
line(x,y,x1,y1,c)
Where x,y is the coordinate of one end of the line, and x1,y1 is the other end.
That's enough for now. I have the basics of animating text as well as basic shapes. Next time I'll dive into PICO-8's sprite editor and creating and animating sprites.
You can pull my cart here and run it if you want.
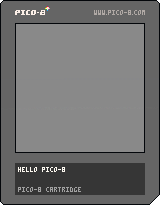
See you next time.