Animating Sprites
Now that we know how to animate text and simple shapes and how to use the Sprite Editor we have enough knowledge to animate a sprite. Let's animate!
The Sprites
I'm going to keep it simple; I'm a simple guy. So that is what I'm going to animate–a simple guy. I created two sprites that show a person running. As I said, I'm not an artist–this is the best I can do. We'll flip between each of the two images to achieve the running. I hope.
Here is a shot of the two sprites I'll be using.
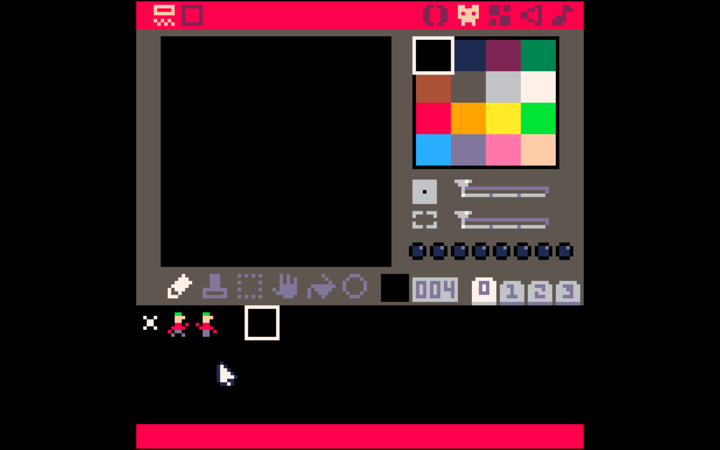
And a closeup of one.
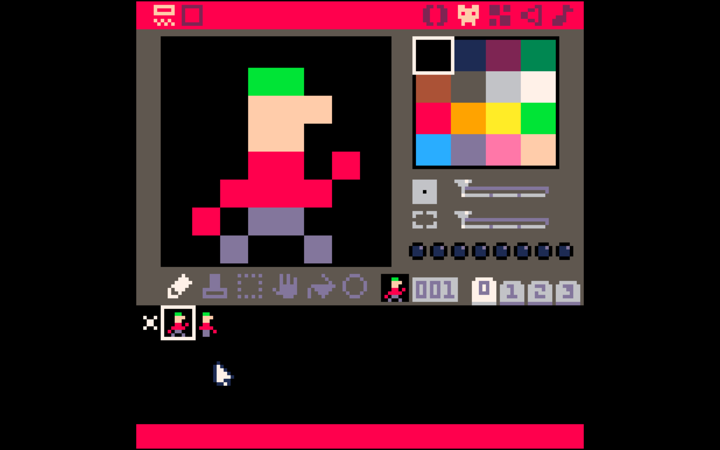
It sort of looks like Ferb from the Disney show, Phineas and Ferb.
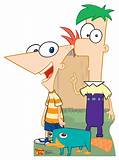
Anyway, now that we have our sprites I can use the program I introduced in the previous post which animates shapes to move "Ferb". I'll make some adjustments to the code.
The Code
First, we want to have him run along the bottom of the screen, so I'll get rid of the vertical component of the animation. I'll also set the initial y component near the bottom of the screen. The last thing I need to do is replace the drawPixel with a function to draw the sprite. The command to draw a sprite is:
spr(n,x,y,)
where n is the sprite number. I'll be using sprites 1 and 2. x and y are the coordinates. I'll update the drawPixel function accordingly. Here is the modified code.
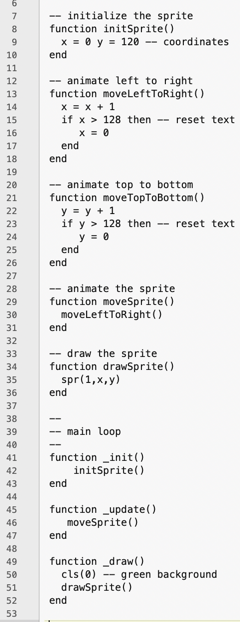
And this is what it looks like when it runs. Click the image to view the animation.
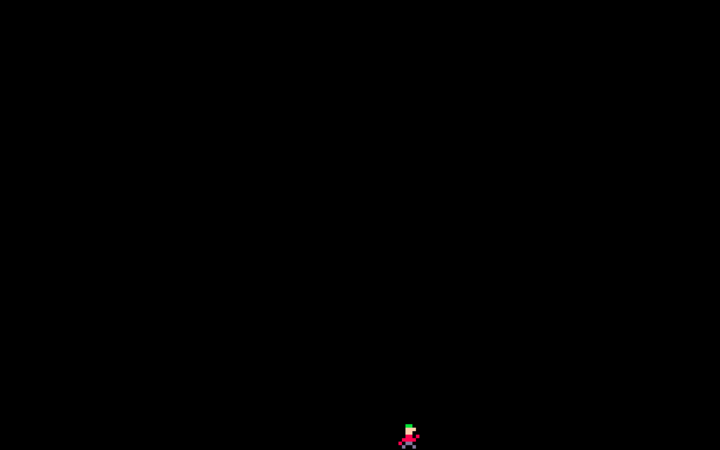
This works nicely, but it's not much of an animation. Let's flip between the two sprites instead of just displaying sprite number 1. I'll do this in the _update function. I will need to keep track of which sprite is being currently displayed so I'll need to set a variable in _init.
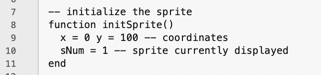
I'll use the new variable sNum in drawSprite instead of hard-coding sprite number 1.
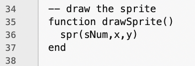
Finally, I'll update sNum after each frame to use the other frame.
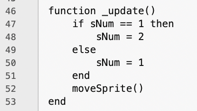
That's all I should need. And this is what it looks like when I run it. Click the image to see the animation.
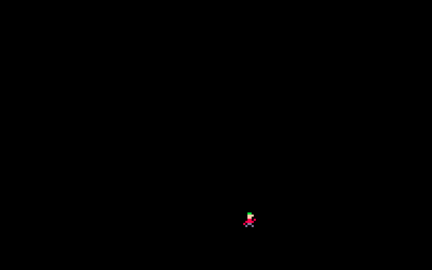
Woah! It works, but Ferb is manic. I need to slow him down. Remember, PICO-8 animates at 30fps so he flaps his arms 30 times per second. Digging through the PICO-8 commands, I don't see a way to delay execution. It looks like I'll have to "roll my own".
Delaying
The easiest way to do this is to delay switching between the sprites in the _update function. I can count the number of frames to skip before we switch. I'll need a variable to keep track of frame counts. I'll put this in _init and update and check it in _update.
Here is the updated _init function.
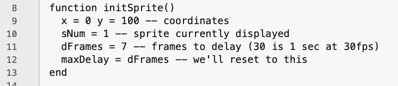
And the new delay function.
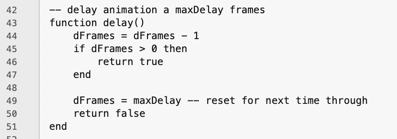
I decrement my dFrames variable and see if it hits 0 which means it's time to change sprites. If not, I just return. If it hits 0 I need to reset the variable to my original delay so I can process the next animation loop.
And, finally, I use delay in our _update function.
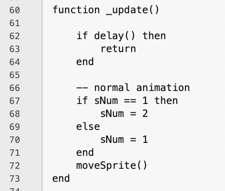
I just call my delay function each time through update and see if it has hit 0, if not I don't do anything in _update, otherwise I switch which sprite to display. This is what it looks like animating. (Click the image again).
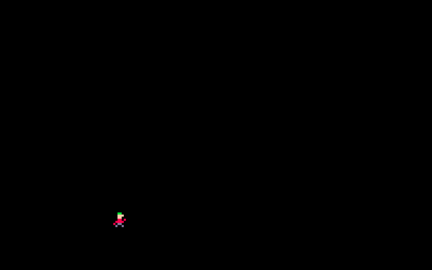
This is switches sprites every 7 frames. You can adjust the variable dFrames in _init to make it faster of slower.
I'm sure there are better more elegant solutions to adjusting the animation speed, but this is the first method that I came up with. It's simple and it works.
Next time, I'll work on controlling Ferb using the keyboard.